实机演示
链接:https://pan.baidu.com/s/16y5Osxkc0p_2nkv1_Gap7g
提取码:2m7d
源码&可执行程序
头文件Cal.h
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| #ifndef __CAL_H__ #define __CAL_H__
#include <stdio.h> #include <stdlib.h> #define Capacity 114514
typedef struct Number { float Data[Capacity]; int top; }number;
typedef struct FuHao { char Data[Capacity]; int top; }fuhao;
number Numbers; fuhao FuHaos;
void InitialNum(number *ra); void InitalFuHao(fuhao *op);
int ClearNumber(number *ra); int ClearFuHao(fuhao *op);
int Pushnumber(number *ra,float e); int Pushfuhao(fuhao *op,char e);
int Popnumber(number *ra,float *e); int Popfuhao(fuhao *op,char *e);
float PeekTopNumber(number *ra); char PeekTopfuhao(fuhao *op);
int IsValid(char ch);
int Level(char s);
int LevelCompare(char op1,char op2);
float CalculateI(float a,float b,char c);
float CalculateII(number *ra,fuhao *op); #endif
|
这个文件“Cal.c”我放了主要函数的内容
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222
| #include "Cal.h"
void InitialNum(number *ra) { ra->top = -1; } void InitalFuHao(fuhao *op) { op->top = -1; }
int ClearNumber(number *ra) { ra->top = -1; return 0;
} int ClearFuHao(fuhao *op) { op->top = -1; return 0; }
int Pushnumber(number *ra,float e) { if(ra->top == Capacity-1) return 0; ra->top++; ra->Data[ra->top] = e; return 1; } int Pushfuhao(fuhao *op,char e) { if(op->top == Capacity-1) return 0; op->top++; op->Data[op->top] = e; return 1; }
int Popnumber(number *ra,float *e) { if(ra->top == -1) return 0; *e = ra->Data[ra->top]; ra->top--; return 1; } int Popfuhao(fuhao *op,char *e) { if(op->top == -1) return 0; *e = op->Data[op->top]; op->top--; return 1; }
float PeekTopNumber(number *ra) { if(ra->top == -1) return 0; return ra->Data[ra->top]; } char PeekTopfuhao(fuhao *op) { if(op->top == -1) return 'N'; return op->Data[op->top]; }
int IsValid(char ch) { if(ch == '(' || ch == ')' || ch == '+' || ch == '-' || ch == '*' || ch == '/' || ch == '=') return 1; return 0; }
int Level(char s) { switch(s) { case '(': return 4; case '*': case '/': return 3; case '+': case '-': return 2; case ')': return 1; default: return 0; } }
int LevelCompare(char op1,char op2) { if(Level(op1) < Level(op2)) return 0; return 1; }
float CalculateI(float a,float b,char c) { switch(c) { case '+': return a+b; case '-': return a-b; case '*': return a*b; case '/': if(b == 0) exit(1); return a/b;
} }
float CalculateII(number *ra,fuhao *op) { float a,b; char ch,s; Pushfuhao(op,'='); printf("请输入计算式(键入等号后回车以表示输入完成):"); ch = getchar(); while(ch != '=' || PeekTopfuhao(op) != '=') {
if(!IsValid(ch)) { int topT = 0; char xx[999]; for(int i = 999;i>=topT;i--) { xx[i] = 0; } while(!IsValid(ch)) { xx[topT] = ch; topT++; ch = getchar(); if(topT>=999) { exit(0); } } float tempT; tempT = atof(xx); Pushnumber(ra,tempT); } else { if(PeekTopfuhao(op) == '(') { if(ch == ')') Popfuhao(op,&s); else Pushfuhao(op,ch); ch = getchar(); } else { if(!LevelCompare(PeekTopfuhao(op),ch)) { Pushfuhao(op,ch); ch = getchar(); } else { Popnumber(ra,&b); Popnumber(ra,&a); Popfuhao(op,&s); Pushnumber(ra,CalculateI(a,b,s)); } } } } printf("结果是:%f\n",PeekTopNumber(ra)); return 0; }
|
这个文件是“main.c”文件,程序的入口
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| #include <stdio.h> #include <stdlib.h> #include "Cal.h" #include "Cal.c" int main() { InitialNum(&Numbers); InitalFuHao(&FuHaos); while(114514) { CalculateII(&Numbers,&FuHaos); ClearNumber(&Numbers); ClearFuHao(&FuHaos); } return 0; }
|
链接:https://pan.baidu.com/s/15pkHOv0KHqH50FUSNgmV3g
提取码:6wlh
内含源码和可执行程序
函数流程图
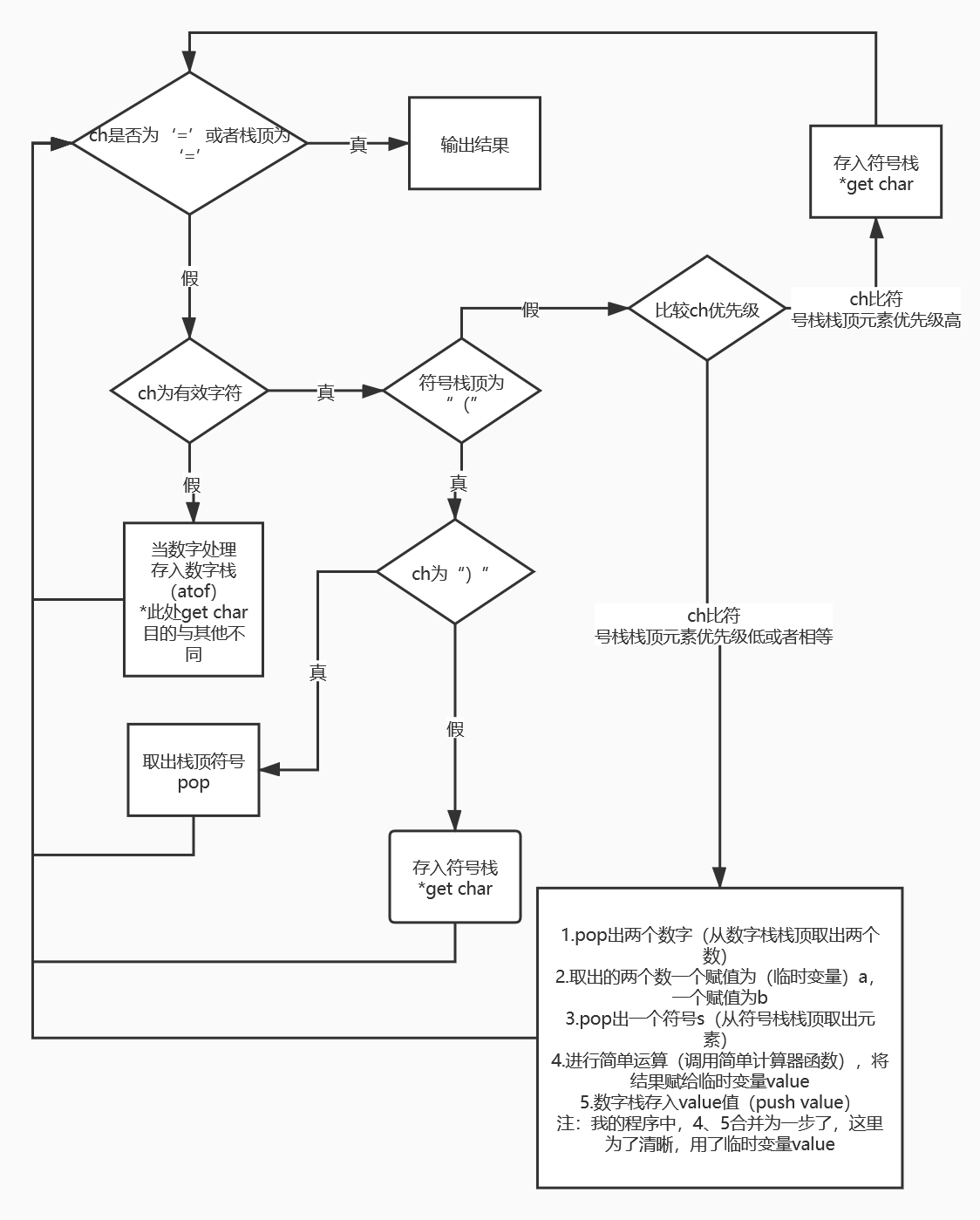
视频解说
链接:https://pan.baidu.com/s/1YF8-y15lb6M82FGxNWdPug
提取码:bd2c
几个口误的地方:
讲解视频上:12分40秒左右
并不是“是需要”或者说是“必须”。这里严格意义上来说没有说正确。具体的栈使用情况还需要看具体的情况。我这里说的终止符首位相碰,是针对我写的这个程序而言的。
讲解视频下:26:31
此处口误,除号优先级比等号要高,所以存入符号栈